A wash trade is a form of market manipulation in which an investor simultaneously sells and buys the same financial instruments to create misleading, artificial activity in the marketplace First, an investor will place a sell order, then place a buy order to buy from themselves, or vice versa.
In the NFT market, wash trades can largely affect the market performance of an item. Speculators can profit by pulling up the trading volume through wash trades, creating FOMO sentiment, which in turn can lure other traders to buy.
Therefore, if you need to obtain and analyze more realistic trading data, we offer the option to exclude wash trades from our endpoints for you to choose from freely.
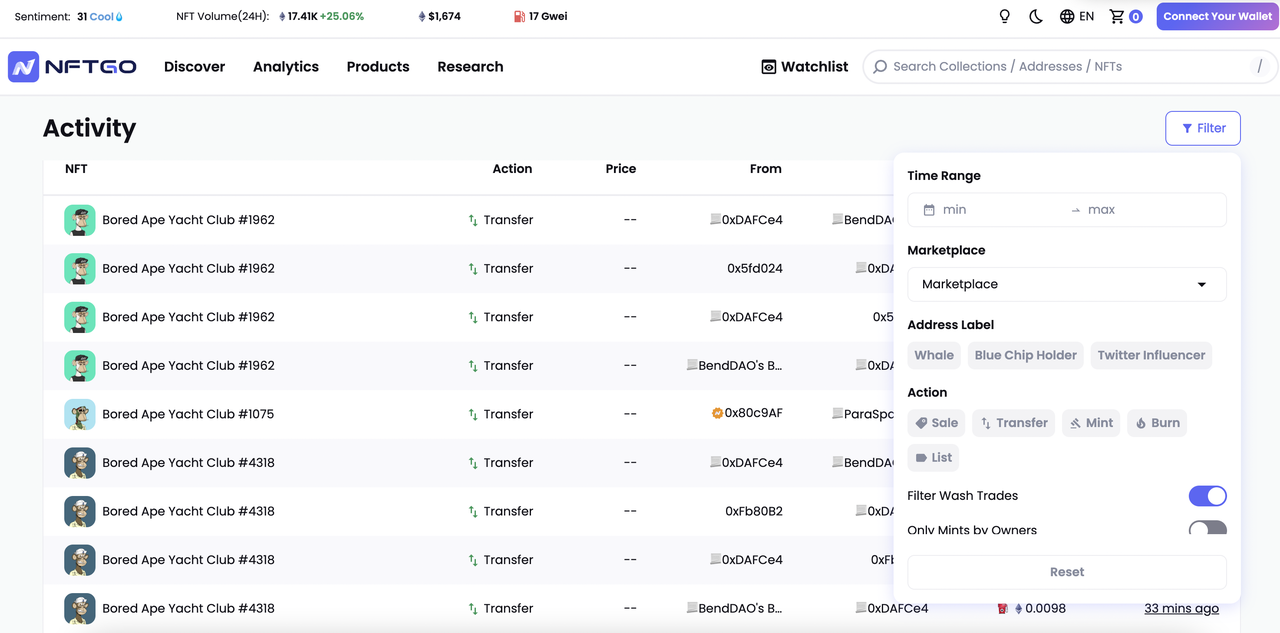
Retrieve collection transaction history
To call this endpoint, you need to fill in following parameters
- contract_address (string): Address of the contract for an NFT collection, beginning with 0x.
- event (string): The type of transactions, including mint, sale, transfer, burn, and all.
- start_time(date-time): Queries can be bounded by a Start Time and End Time. Start Time is the earliest timestamp to include in the transaction query, formatted as UTC timestamp in seconds or ISO 8601 format date-time string, such as 2022-08-20T00:00:00+00:00. Start Time limited to 2021-09-01T00:00:00+00:00 or later.
- end_time(date-time): Where an End Time is specified, transaction queries will fetch all transactions up to but excluding the End Time, which is formatted as UTC timestamp in seconds or ISO 8601 format date time string. The query window varies according to the plan.
- offset (integer): The size of a returned data segment.
- asc (boolean): Whether to sort results in ascending order.
- exclude_wash_trading (boolean): Whether excluding wash trading transactions.
And add the following code to the main.js
import fetch from 'node-fetch';
// Replace with your NFTGo API key:
const apiKey = "YOUR-API-KEY";
const options= {
method: 'GET',
headers: {
accept: 'application/json',
'X-API-KEY': apiKey
}
};
// Replace with the contract address you want to query:
const contractAddress = "0xbc4ca0eda7647a8ab7c2061c2e118a18a936f13d";
// Replace with the event you want to query:
const event= "all";
// Replace with the start time you want to query:
const startTime= "2022-08-20T00:00:00+00:00";
// Replace with the end time you want to query:
const endTime= "2022-09-01T00:00:00+00:00";
// Replace with the offset you want to query:
const offset = 0;
// Replace with the limit you want to query:
const limit = 20;
// Replace with the asc you want to query:
const asc= false;
// Replace with the exclude_wash_trading you want to query:
const excludeWashTrading= false;
const url = `https://data-api.nftgo.io/eth/v1/history/collection/${contractAddress}/transactions?event=${event}&start_time=${startTime}&end_time=${endTime}&offset=${offset}&limit=${limit}&asc=${asc}&exclude_wash_trading=${excludeWashTrading}`;
// Make the request and print the formatted response:
fetch(url, options)
.then(res => res.json())
.then(json => console.log(json))
.catch(err => console.error('error:' + err));
Your output should look like this.
{
"total": 10000,
"transactions": [
{
"blockchain": "ETH",
"sender": {
"address": "0xa5004c8b2d64ad08a80d33ad000820d63aa2ccc9",
"is_whale": false,
"blockchain": "ETH",
"is_contract": "False",
"is_blue_chip_holder": "False"
},
"receiver": {
"address": "0xd2dc28f65c76510a4a930d28664a60828c4cf64e",
"ens": "tima.eth",
"is_whale": false,
"blockchain": "ETH",
"is_contract": "False",
"is_blue_chip_holder": "False"
},
"event": "transfer",
"quantity": 1,
"gas_fee_usd": 5.0716,
"tx_hash": "0x286b7537cc79dca661b28fc58137b9f0385d1e3886a147e087922e104d1d4311",
"time": 1652626903,
"nft": {
"contract_address": "0xbc4ca0eda7647a8ab7c2061c2e118a18a936f13d",
"token_id": "6512",
"name": "Bored Ape Yacht Club #6512",
"blockchain": "ETH"
},
"gas_fee": {
"quantity": 0.006,
"value": 0.006,
"crypto_unit": "ETH",
"usd": 11.643
},
"price": {
"quantity": 86.8042,
"value": 86.8042,
"crypto_unit": "ETH",
"usd": 99614.18167703273
}
}
]
}